CO4403 Coursework 1: Development Task
THE BRIEF/INSTRUCTIONS
This assignment consists of four parts, you must work through them in order (start with design then attempt development etc). You must achieve a mark of at least 50% to pass this assignment. It is very important that you work on this coursework by yourself and do not let other students see or use your code. Your work will be marked through a video demo you create and an assessment of your code and UML. Your mark will be comprised of four parts:
Design Part (UML Class Diagram) 15% Development Part (Java Code) 30% Video Demo Part (Video with Audio) 15% UI and File IO Part (Java Code) 40% Learning outcomes assessed1. Explain and use key programming constructs to create correct and efficient programs 2. Design, and implement Object-Oriented programs 3. Develop applications with graphical user-interfaces
1. Design Part– 15% Write your full name down the ‘A’ column in the table below one character in each row omitting spaces and punctuation. If your full name is not long enough just repeat it. The combination of column A and B give you the names of classes and methods to use in your assignment. For example, if your name was ‘Daniel …’ this would give you DParentClass, aChild1Class, nChild2Class etc
Using the information from the table above hand-draw a UML Class diagram on paper, ensure that: · you use the correct notation for relationships between classes, · class names are correct, · method names are correct, · method parameters/return types are correct, · class instance variables are correct, · variables are named appropriately, · access modifiers are shown where appropriate, you are free to choose which modifier to apply where this is not mentioned in the table above. You do not need to include class constructors in your UML diagram. Take a photo of your UML diagram and submit it through Blackboard along with the table above.
2. Development Part – 30% You need to work through the following series of tasks in order; your mark will depend on your progress and the quality of the work you have done. You only need to submit your finished code after you have completed all tasks.
Task 1: Implement the UML Class diagram you have created in Java code. Ensure the classes are in separate files.
Task 2: Create a driver program that creates instances of all classes, calls all methods with appropriate parameters, and outputs the results to the console.
Task 3: Add code into each method to act on the parameters and use them to generate an appropriate return value using your knowledge of the Java language. You need to decide how to do this based on the parameters and return type of a method. For example, if given ‘3x String as parameters, returns a double’ you could convert all Strings to doubles (using Double.parseDouble or similar) and then return the highest.
Task 4: Add constructor methods to each of the 3 classes and use them to initialize instance variables.
Task 5: Extend Task 3 so the class instance variables are acted upon (i.e. data added and retrieved) in at least one method in each class.
Task 6: Ensure your driver program demonstrates that you have completed all the tasks, particularly that the methods act on given parameters (Task 3) and that methods act upon class instance variables (Task 5). You will be expected to submit your entire source code (and Eclipse project). Your code must be commented, and you must include a separate document of references to any resources you used in the development of your application (books, web sites, forums etc).
3. Video Demo Part – 15%
Once your development is complete you must create a video demonstration which explains and justifies how you implemented Task 3 and Task 5 using the output of your driver program (or User Interface if you attempt part 4) to demonstrates that your code works successfully. This should be a screen capture video with voice-over of your explanation. This demo must be no longer than 5 minutes. Screen capture software is available free to all students through Office 365 using Microsoft Stream (https://web.microsoftstream.com/). Separate instructions will be uploaded to Blackboard on how to use this. The video demo is critical part of the assignment where you show how you have met the marking criteria. Please ensure that both the audio and visuals are clear in your video. Your video file must be submitted in a format that can be accessed easily by your marker.
4. User Interface (UI) and File IO Part – 40% Create a User Interface in SWING based on the material covered on the module. Do not use Java FX and do not use any GUI builder tools. This should include three JFrames, one must be menu which is displayed when the program starts with JButtons for three options: 1) Test Child1Class 2) Test Child2Class, 3) Exit. For options 1 and 2 you need to display a JFrame that provides a way for a user to test all three of the methods shown in the table in the design part ie for each there should be appropriate JTextFields for the user to input the data to be passed into the methods as parameters, a ‘Test’ button which if clicked calls the method (having converted the parameters in the JTextFields to appropriate types) and show the result on the user interface. Every time a test is carried out you should log to a file called TestLog.txt a new line beginning with a timestamp (current date and time), the method that was tested, the parameters passed in and the output generated. If you have completed this part of the assignment then show your user interface instead of your driver program in the video demo. Marking Criteria
1. Design Part (Worth 15% of this Assignment) To obtain 5% you must have produced something recognizable as a UML class which aligns with the brief which is hand-drawn. You must also submit the completed table from part 1 of this assignment brief. To obtain 10%, in addition to the above, you will have produced a correctly constructed hand-drawn UML diagram showing relationships between classes and correct class/method names with only very minor mistakes. To obtain 15%, in addition to the above, you will have produced a correctly constructed hand-drawn UML diagram correctly showing relationships between classes and correct class/method names with no mistakes.
2. Development Part (Worth 30% of this Assignment) To obtain 10% you must have completely implemented your given UML diagrams into Java objects, your code must compile and have a driver program which creates objects and calls methods. To obtain 20%, in addition to the above, you must have completed Task 3 successfully (with only very minor mistakes) and evidenced application of key Java programming constructs (such as if conditional or for loops). To obtain 30%, in addition to the above, you must have completed all tasks successfully (with only very minor mistakes). Implementations of tasks 3 and 5 will show understanding and application of key Java programming constructs (iteration/selection) in appropriate ways. Your source code will be appropriately organized and commented.
3. Demo Part (15% of this Assignment) To obtain 10% for the demo you must have demonstrated a working and interactive Java SE application conforming to the assignment specification and clearly explained key technical aspects of Java used.
To obtain 15% for the demo, in addition to the above, you must have clearly and competently described the code applied in the implementation tasks 3 and 5.
4. User Interface and File IO Part (40% of this Assignment) To obtain 10%, you must have successfully implemented the menu with 3 options and made an attempt to implement both JFrames which test the classes, the user interfaces must be complete but may not work properly. To obtain 20%, in addition to the above, the JFrames which test the classes will be partly functional and call the appropriate methods with information entered by the user in the user interface. To obtain 30%, in addition to the above, the JFrames which test the classes will be implemented to a high stand and work without any errors. To obtain 40%, in addition to the above, the program will log all testing of the classes to the file as described in the assignment brief without any omissions or errors. Late workLate work can be submitted within 5 working days of the deadline for a maximum grade of 50%. Except where an extension of the hand-in deadline date has been approved, work submitted after this time will receive a mark of 0%. |
|||||||||||||||||||||||||||||||||||||
PREPARATION FOR THE ASSESSMENT
The preparation for this assignment was provided in the lecture materials and associated activities in weeks 1-7 of semester 1. |
|||||||||||||||||||||||||||||||||||||
RELEASE DATES AND HAND IN DEADLINE
Assessment Release date: 8th November 2021 Assessment Deadline Date and time: 5pm on 17th December 2021. Please note that this is the final time you can submit – not the time to submit! Do not contact module tutors to request an ad-hoc extension, such requests will always be will be refused. If you feel you need to apply for an extension/have mitigating circumstances you need to follow the correct university process detailed at https://www.uclan.ac.uk/students/support/extensions.php Your feedback and mark for this assessment will be provided within 15 working days after submission |
|||||||||||||||||||||||||||||||||||||
SUBMISSION DETAILS You must submit all design, source code and video demo parts of the assignment. If you fail to submit all parts or submit them in a form which cannot be accessed by module tutors your will receive a mark of 0. You must submit 1) a video demo (a screen capture with a clear voice-over explanation) which shows your application working successfully and where you have met the marking criteria. Your video must be no longer that 5 minutes, and you must upload your video file to Blackboard. 2) your entire Eclipse project in a .zip file via the appropriate link on Blackboard. 3) all your code inside a text document (e.g. Word document or plain text document) via the appropriate link on Blackboard. 4) Your UML class diagram (drawn by hand and photographed) via the appropriate link on Blackboard.
If you do not submit all parts of the assignment in a form which can be accessed by module tutors you will receive a mark of 0. |
|||||||||||||||||||||||||||||||||||||
HELP AND SUPPORT
· Assignment support will be provided in the CO4403 Microsoft Team during scheduled lab hours. · For support with using library resources, please contact SubjectLibrarians@uclan.ac.uk. You will find links to lots of useful resources in the My Library tab on Blackboard. · If you have not yet made the university aware of any disability, specific learning difficulty, long-term health or mental health condition, please complete a Disclosure Form. The Inclusive Support team will then contact to discuss reasonable adjustments and support relating to any disability. For a copy of the form and more information, visit the Inclusive Support site. · To access mental health and wellbeing support, you can email wellbeing@uclan.ac.uk, call 01772 893020 or visit our UCLan Wellbeing Service pages for more information. · If you have any other query or require further support you can contact Student Support in the Student Centre. Speak with us for advice on accessing all the University services as well as the Library services. Whatever your query, our expert staff will be able to help and support you. For more information, how to contact us and our opening hours visit https://msuclanac.sharepoint.com/sites/StudentHub/SitePages/Contact-Us.aspx · If you have any valid mitigating circumstances that mean you cannot meet an assessment submission deadline and you wish to request an extension, you will need to apply online prior to the deadline. |
|||||||||||||||||||||||||||||||||||||
Disclaimer: The information provided in this assessment brief is correct at time of publication. In the unlikely event that any changes are deemed necessary, they will be communicated clearly via e-mail and a new version of this assessment brief will be circulated. | Version: 1 |
Expert's Answer
Chat with our Experts
Want to contact us directly? No Problem. We are always here for you
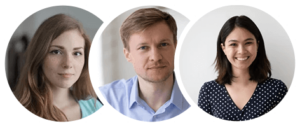
Get Online
Online Tutoring Services